Sstf Disk Algorithm Coding
I need a C program to simulate disk scheduling algorithms. It's my assignment (due tomorrow). These algorithms need to be simulated: FCFS, SSTF, SCAN, LOOK, C-SCAN and C-LOOK. Test your code with the data. They are fairly small. Lot of data/pre-written code examples are already there if you look on google. A simple implementation of FCFS and SSTF disk scheduling algorithms in Python. Python fcfs sstf disk-scheduling-algorithms Updated Mar 4, 2020. Program to implement SSTF disk scheduling algorithm /. Variable description: req - array for taking the request, index - array fo.
- Sstf Disk Scheduling Algorithm Program In Java
- Sstf Disk Algorithm Coding Guide
- Sstf Disk Algorithm Coding Instructions
Let us learn how to implement shortest seek time first algorithm in C programming with its explanation, output, advantages, disadvantages and much more.
What is Shortest Seek Time First Disk Scheduling Algorithm?
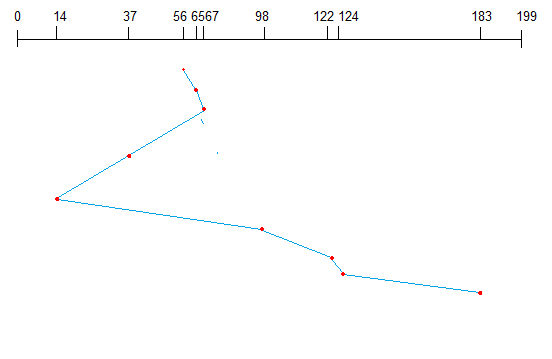
The shortest seek time first is commonly abbreviated as SSTF. It is also popularly known as shortest seek first algorithm.
The SSTF disk scheduling algorithm is a secondary storage scheduling algorithm which enables to determine the motion of the disk’s arm and the disk head in servicing read and write requests.
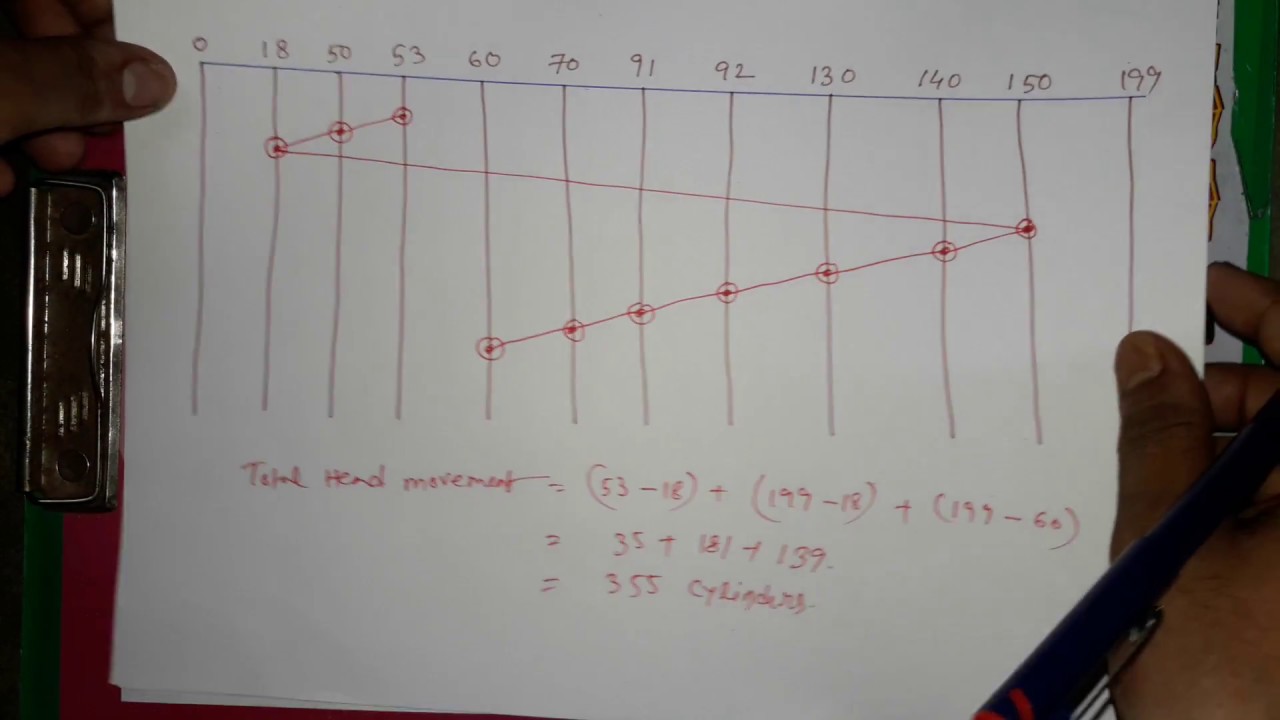
This algorithm helps to determine which job is nearest to the current head position with minimum seek time, and then services that job next.
In the SSTF algorithm, the jobs having the shortest seek time are executed first. Therefore, the seek time of each job is pre-calculated in the job queue and every job is scheduled according to its seek time.
This enables the job nearest to the disk arm to get executed first. It also has a better average response time and throughput as compared to the FCFS scheduling algorithm.
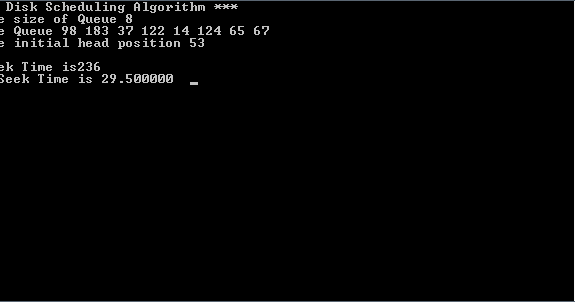
Sstf Disk Scheduling Algorithm Program In Java
Advantages
- Reduced total seek time as compared to FCFS algorithm
- Increased throughput time
- Decreased average response time
Disadvantages
- There are high chances of starvation if the seek time is higher than the incoming jobs
- Switching the directions may slow down the process
- There are chances of overheads.
- This algorithm is not the most optimal one
Note: This C program to implement SSTF algorithm is compiled with GNU GCC compiler using CodeLite IDE on Microsoft Windows 10 operating system.
C Program For Shortest Seek Time First Disk Scheduling Algorithm
2 4 6 8 10 12 14 16 18 20 22 24 26 28 30 32 34 36 38 40 42 44 46 48 50 52 54 56 58 60 62 64 66 68 70 72 74 76 | { intflag; { intarray_1[33],array_2[33]; intcount=0,j,x,limit,minimum,location,disk_head,sum=0; scanf('%d',&limit); scanf('%d',&disk_head); while(count<limit) scanf('%d',&h[count].num); count++; for(count=0;count<limit;count++) x=0; location=0; { { { if(array_1[j]<0) array_1[j]=h[j].num-disk_head; minimum=array_1[j]; x++; else array_1[j]=disk_head-h[j].num; { } if(minimum>array_1[j]) minimum=array_1[j]; } } array_2[count]=h[location].num-disk_head; { } } while(count<limit) sum=sum+array_2[count]; } printf('nTotal movements of the cylinders:t%d',sum); } |
Output
Sstf Disk Algorithm Coding Guide
If you have any doubts about the implementation of shortest seek first disk scheduling C program, let us know about it in the comment section. Find more about it here.
Sstf Disk Algorithm Coding Instructions
CPU Scheduling Algorithms |
---|
FCFS Algorithm |
Preemptive Shortest Job First Algorithm |
Round Robin Algorithm |
Shortest Job First Algorithm |
C SCAN Scheduling C Program |
SCAN Algorithm |
Multi-Level Feedback Queue Algorithm |
Preemptive Priority Algorithm |
Priority Scheduling Algorithm |
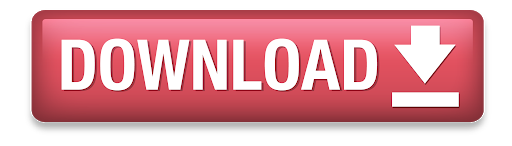